Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Wednesday, November 30, 2011
Conway's Game of Life in XNA
The following video demonstrates an implementation of Conway's Game of Life I once wrote in in XNA.
Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Monday, November 7, 2011
Cheating at a typing test with JavaScript
So I just found this website called 10fastfingers and it has a typing game that measures your speed in WPM. I played with it for a while to see how fast I could really type but after I got bored keying in random words in a text box, I decided to spice things up a bit...by cheating; because hey, why not?
And so, I wrote two scripts to do the typing for me; sort of like secretaries. The first one just completes the test instantly, which guarantees that all the words available in the test are "typed in". The second one takes its time with a delay between each word, but it's more fun to look at because you actually get to see the words which are being completed.
This script is not very entertaining since it finishes the test almost instantly because it's using a for loop to go through the words.
This is a very slightly modified version of the first script which inserts a delay between each word that's "typed in". The delay, in milliseconds, is specified as an argument to the go function.
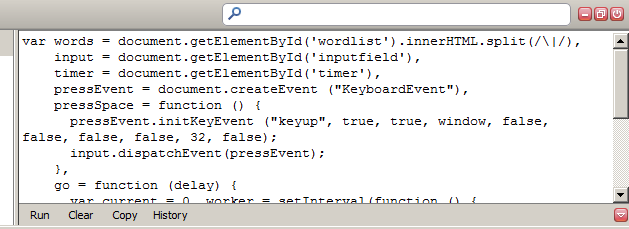
Now press CTRL+ENTER and watch the magic happen.
And so, I wrote two scripts to do the typing for me; sort of like secretaries. The first one just completes the test instantly, which guarantees that all the words available in the test are "typed in". The second one takes its time with a delay between each word, but it's more fun to look at because you actually get to see the words which are being completed.
The instant one
var words = document.getElementById('wordlist').innerHTML.split(/\|/), input = document.getElementById('inputfield'), pressEvent = document.createEvent("KeyboardEvent"), pressSpace = function () { pressEvent.initKeyEvent("keyup", true, true, window, false, false, false, false, 32, false); input.dispatchEvent(pressEvent); }, go = function () { var i = 0, j = words.length; for (; i < j; ++i) { input.value += words[i]; pressSpace(); } }; go();
This script is not very entertaining since it finishes the test almost instantly because it's using a for loop to go through the words.
Putting a delay
var words = document.getElementById('wordlist').innerHTML.split(/\|/), input = document.getElementById('inputfield'), timer = document.getElementById('timer'), pressEvent = document.createEvent("KeyboardEvent"), pressSpace = function () { pressEvent.initKeyEvent("keyup", true, true, window, false, false, false, false, 32, false); input.dispatchEvent(pressEvent); }, go = function (delay) { var current = 0, worker = setInterval(function () { if (current == words.length || timer.className) { clearInterval(worker); return; } input.value += words[current++]; pressSpace(); }, delay); }; go(60000 / words.length);
This is a very slightly modified version of the first script which inserts a delay between each word that's "typed in". The delay, in milliseconds, is specified as an argument to the go function.
Running the scripts
To run the scripts, open Firefox, head over to to http://speedtest.10-fast-fingers.com/, launch Firebug and paste the script in the console.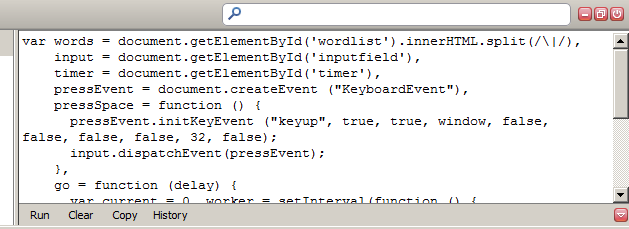
Now press CTRL+ENTER and watch the magic happen.
Posted by
Andreas Grech
at
9:39 PM
Cheating at a typing test with JavaScript
2011-11-07T21:39:00+01:00
Andreas Grech
Javascript|
Comments


Labels:
Javascript
Subscribe to:
Posts (Atom)