Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Wednesday, November 30, 2011
Conway's Game of Life in XNA
The following video demonstrates an implementation of Conway's Game of Life I once wrote in in XNA.
Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Unfortunately, I currently don't have the source code for this online but I'll post it once I stop procrastinating.
Monday, November 7, 2011
Cheating at a typing test with JavaScript
So I just found this website called 10fastfingers and it has a typing game that measures your speed in WPM. I played with it for a while to see how fast I could really type but after I got bored keying in random words in a text box, I decided to spice things up a bit...by cheating; because hey, why not?
And so, I wrote two scripts to do the typing for me; sort of like secretaries. The first one just completes the test instantly, which guarantees that all the words available in the test are "typed in". The second one takes its time with a delay between each word, but it's more fun to look at because you actually get to see the words which are being completed.
This script is not very entertaining since it finishes the test almost instantly because it's using a for loop to go through the words.
This is a very slightly modified version of the first script which inserts a delay between each word that's "typed in". The delay, in milliseconds, is specified as an argument to the go function.
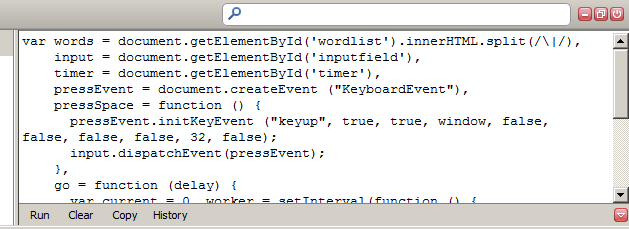
Now press CTRL+ENTER and watch the magic happen.
And so, I wrote two scripts to do the typing for me; sort of like secretaries. The first one just completes the test instantly, which guarantees that all the words available in the test are "typed in". The second one takes its time with a delay between each word, but it's more fun to look at because you actually get to see the words which are being completed.
The instant one
var words = document.getElementById('wordlist').innerHTML.split(/\|/), input = document.getElementById('inputfield'), pressEvent = document.createEvent("KeyboardEvent"), pressSpace = function () { pressEvent.initKeyEvent("keyup", true, true, window, false, false, false, false, 32, false); input.dispatchEvent(pressEvent); }, go = function () { var i = 0, j = words.length; for (; i < j; ++i) { input.value += words[i]; pressSpace(); } }; go();
This script is not very entertaining since it finishes the test almost instantly because it's using a for loop to go through the words.
Putting a delay
var words = document.getElementById('wordlist').innerHTML.split(/\|/), input = document.getElementById('inputfield'), timer = document.getElementById('timer'), pressEvent = document.createEvent("KeyboardEvent"), pressSpace = function () { pressEvent.initKeyEvent("keyup", true, true, window, false, false, false, false, 32, false); input.dispatchEvent(pressEvent); }, go = function (delay) { var current = 0, worker = setInterval(function () { if (current == words.length || timer.className) { clearInterval(worker); return; } input.value += words[current++]; pressSpace(); }, delay); }; go(60000 / words.length);
This is a very slightly modified version of the first script which inserts a delay between each word that's "typed in". The delay, in milliseconds, is specified as an argument to the go function.
Running the scripts
To run the scripts, open Firefox, head over to to http://speedtest.10-fast-fingers.com/, launch Firebug and paste the script in the console.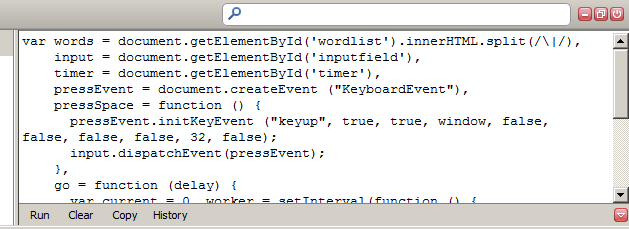
Now press CTRL+ENTER and watch the magic happen.
Posted by
Andreas Grech
at
9:39 PM
Cheating at a typing test with JavaScript
2011-11-07T21:39:00+01:00
Andreas Grech
Javascript|
Comments


Labels:
Javascript
Wednesday, October 26, 2011
A Chrome extension for plotting graphs with Wolfram|Alpha
This weekend I got round to writing a Google Chrome extension that aids you in plotting graphs from Wolfram|Alpha. The extension simply takes the parameters you input, constructs a url to Wolfram|alpha and then opens a new tab with that url.
If you wanna give it a go, I published it on the Chrome Web Store.
I've also put the source up at github: https://github.com/dreasgrech/wolframalpha-graphplotter
If you wanna give it a go, I published it on the Chrome Web Store.
I've also put the source up at github: https://github.com/dreasgrech/wolframalpha-graphplotter
Posted by
Andreas Grech
at
6:20 PM
A Chrome extension for plotting graphs with Wolfram|Alpha
2011-10-26T18:20:00+02:00
Andreas Grech
Google Chrome Extensions|Javascript|
Comments


Labels:
Google Chrome Extensions,
Javascript
Sunday, October 9, 2011
Finding a function's inverse with Wolfram|Alpha
To check my answers when calculating the inverse of functions, I've found this handy widget from Wolfram|Alpha which does the job:
Posted by
Andreas Grech
at
1:43 PM
Finding a function's inverse with Wolfram|Alpha
2011-10-09T13:43:00+02:00
Andreas Grech
Mathematics|
Comments


Labels:
Mathematics
Wednesday, October 5, 2011
A Function Transformations reference sheet
Since I never miss a change to procrastinate, I decided to spend some time compiling a "cheat sheet" for function transformations.
I've included the following 8 transformations:
I've included the following 8 transformations:
- A constant added to or subtracted from a function shifts its graph vertically.
- A constant added to or subtracted from a function's input shifts its graph horizontally.
- Multiplying a function by a constant stretches or squishes the function vertically.
- Multiplying a function's input by a constant stretches or squishes the function horizontally.
- Multiplying a function by a negative number causes its graph to reflect over the x-axis.
- Multiplying a function's input by a negative number causes its graph to reflect over the y-axis.
- Taking the absolute value of a function moves all the points on its graph above the x-axis.
- Taking the absolute value of a function's input causes the left side of the graph to clone the right side.
Posted by
Andreas Grech
at
7:50 PM
A Function Transformations reference sheet
2011-10-05T19:50:00+02:00
Andreas Grech
Mathematics|
Comments


Labels:
Mathematics
Sunday, October 2, 2011
A State Manager in JavaScript
The following is an implementation for a state manager written in JavaScript. More than one state can be "on" at one moment but mutual exclusivity rules can be set up to allow certain states to not be "on" at the same time i.e. if one of the states is switched on while the other is already on, the latter will be switched off since they can't be both on at the same time.
It also supports two hooks which can be used if you want to be notified when states are changed.
It's implemented with a fluent interface to allow for method-chaining.
The source is here: https://github.com/dreasgrech/statemanager-js
I've set up a simple demo to show how it can be used. You can view it here: http://dreasgrech.com/upload/statemanager-js/demo.html
It also supports two hooks which can be used if you want to be notified when states are changed.
It's implemented with a fluent interface to allow for method-chaining.
The source is here: https://github.com/dreasgrech/statemanager-js
I've set up a simple demo to show how it can be used. You can view it here: http://dreasgrech.com/upload/statemanager-js/demo.html
Basic Usage
var state = stateManager(); state.turnOn("state 1", "state 2"); state.on("state 1"); // true state.on("state 2"); // true state.on("state 3"); // false state.toggle("state 2"); // "state 2" is now off state.on("state 2"); // falsetoggle returns the new state after it's been toggled.
Mutual Exclusivity
var state = stateManager(); state.mutex("state 1", "state 3"); // "state 1" and "state 2" now cannot be both on at the same time state.mutex("state 2", "state 3"); // "state 2" and "state 3" now cannot be both on at the same time state.turnOn("state 1"); state.turnOn("state 3"); // "state 1" will now be turned off since 1 and 3 are mutually exclusive state.turnOn("state 1"); // turning "state 1" on, but switching "state 3" off for the same reason state.turnOn("state 2"); // if "state 3" was on at this point, it would have been switched off
Hooks
var state = stateManager(); state.onStateChanged(function (state, value) { // The global event; fires every time a state changes console.log(state + " is now " + value); }); state.onStateChanged("state 1", function (value) { // The event that will only fire when "state 1" changes console.log("[Custom hook] state 1 has been changed. New value: " + value); }); state.turnOn("state 1"); state.turnOn("state 3", "state 2"); state.toggle("state 1"); state.turnOn("state 2");Output:
state 1 is now 1 [Custom hook] state 1 has been changed. New value: 1 state 3 is now 1 state 2 is now 1 state 1 is now 0 [Custom hook] state 1 has been changed. New value: 0Note that the onStateChanged event fires only when a state changes. For example, if you turnOn("state 2") while "state 2" is already on, the event will not fire. The same goes for when a state is off and you try to turnOff.
Posted by
Andreas Grech
at
8:48 PM
A State Manager in JavaScript
2011-10-02T20:48:00+02:00
Andreas Grech
Javascript|
Comments


Labels:
Javascript
Saturday, October 1, 2011
Function composition in JavaScript
Function composition is the application of a result of a function as input to another function. Say we have the following three functions:
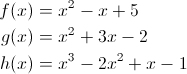
Composing functions f, g and h respectively means that we pass the output from function h as the input to function g and then passing the output from g as the input to function f. Mathematically, it would be represented as follows:

That's essentially the same as:

Functions in JavaScript are first class citizens. This means that they can be stored in variables and data structures, passed as an argument to functions and also returned from functions. This means that we can easily implement function composition in JavaScript with a very trivial function:
Here's an example of how we can use it by composing three functions that represent the three polynomials mentioned at the start of the post:
Composing them together would then be:
The result of the composed function is now the same as the result of f(g(h(x))):
If we had a function f and its inverse f-1 and we compose them, we should get the original input x:
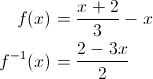

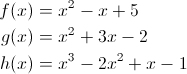
Composing functions f, g and h respectively means that we pass the output from function h as the input to function g and then passing the output from g as the input to function f. Mathematically, it would be represented as follows:

That's essentially the same as:

Functions in JavaScript are first class citizens. This means that they can be stored in variables and data structures, passed as an argument to functions and also returned from functions. This means that we can easily implement function composition in JavaScript with a very trivial function:
Function.prototype.compose || (Function.prototype.compose = function (f) { var g = this; return function () { return g(f.apply(null, arguments)); }; });
Here's an example of how we can use it by composing three functions that represent the three polynomials mentioned at the start of the post:
var f = function (x) { return x*x - x + 5; }, g = function (x) { return x*x + 3*x - 2; }, h = function (x) { return x*x*x - 2*(x*x) + x -1; };
Composing them together would then be:
var composed = f.compose(g).compose(h);
The result of the composed function is now the same as the result of f(g(h(x))):
composed(x) === f(g(h(x))); // true
If we had a function f and its inverse f-1 and we compose them, we should get the original input x:
var f = function (x) { return (x + 2) / 3 - x; }, f_inverse = function (x) { return (2 - 3*x) / 2; }; (f.compose(f_inverse)(x) || f_inverse.compose(f)(x) || x) === x; // true
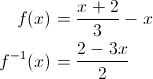

Posted by
Andreas Grech
at
2:17 PM
Function composition in JavaScript
2011-10-01T14:17:00+02:00
Andreas Grech
Javascript|Mathematics|
Comments


Labels:
Javascript,
Mathematics
Thursday, September 29, 2011
JavaScript helpers: Iterating an array while skipping select elements
Array.prototype.iterate || (Array.prototype.iterate = function (callback, ignore) { var i = 0, j = this.length; for (; i < j; ++i) { if (ignore && ignore(this[i], i)) { continue; } callback(this[i], i); } });
Usage
Here's how we can use it to iterate over a couple of numbers, skipping over the even ones:var arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; arr.iterate(function (el) { console.log(el); }, function (el) { return !(el % 2); });
Output:
1 3 5 7 9
Here's another example, this time involving objects:
var queen = function (reference) { return { ref: reference }; }, arr = [queen("Aragon"), queen("Boleyn"), queen("Seymour"), queen("Cleves"), queen("Howard"), queen("Parr")]; arr.iterate(function (el, i) { console.log(i, el.ref); }, function (q) { return q.ref === "Boleyn" || q.ref === "Howard"; });Output (the queens who died with their heads on):
0 Aragon 2 Seymour 3 Cleves 5 Parr
If no ignore function is passed as the second parameter of the iterate method, no elements are skipped:
var arr = ["Mr. Albert Show", "Fusion Orchestra", "Fuzzy Duck", "Minotaurus"]; arr.iterate(function (el, i) { console.log(el); });Output
Mr. Albert Show Fusion Orchestra Fuzzy Duck Minotaurus
Posted by
Andreas Grech
at
7:13 PM
JavaScript helpers: Iterating an array while skipping select elements
2011-09-29T19:13:00+02:00
Andreas Grech
Javascript|
Comments


Labels:
Javascript
Saturday, September 17, 2011
Finding a YouTube video title by scraping
I was recently writing a tool in C# and I needed a way to get a YouTube video title. I could have used the YouTube API but I wasn't bothered about setting everything up and going through the documentation, so I decided to scrape the title off the video page itself.
For this, I first wrote a very simple way to execute a GET request and return the response (Removed all forms of error checking for brevity):
For this, I first wrote a very simple way to execute a GET request and return the response (Removed all forms of error checking for brevity):
public static string ExecuteGETRequest(string url) { var request = (HttpWebRequest)WebRequest.Create(url); using (var response = (HttpWebResponse)request.GetResponse()) { var reader = new StreamReader(response.GetResponseStream()); return reader.ReadToEnd(); } }And here's the method that does the task at hand:
public static string GetYouTubeVideoTitle(string youtubeLinkUrl) { string response = ExecuteGETRequest(youtubeLinkUrl), title = response.Substring(response.IndexOf("<title>\n") + 8); title = title.Substring(0, title.IndexOf("\n")); return title.Trim(); }
Usage
var title = GetYouTubeVideoTitle("http://www.youtube.com/watch?v=TY9jN6hm3N0"); // "Wicked Lady - Ship of Ghosts (Part 1 of 2) 1972"Be wary of using this method for extracting titles because it could potentially break in the future due to changes in how YouTube renders the page with HTML; but it may be useful for quick scripts.
Checking for link validity
If you want to make sure the given link actually points to a YouTube video, you can use something like this:public static bool IsValidYoutubeLink(string youtubeLink) { // Regular expression from http://www.regexlib.com/REDetails.aspx?regexp_id=2569 return Regex.IsMatch(youtubeLink, @"^http://\w{0,3}.?youtube+\.\w{2,3}/watch\?v=[\w-]{11}"); }
Sunday, September 11, 2011
Generating a list of links for a user's YouTube uploads
This is a very simple application I just wrote to generate a list of all of the video upload links given a YouTube user.
Download the exe (release) file from: http://dreasgrech.com/upload/youtubeuseruploads/YouTubeUserUploads.exe
And the source: http://dreasgrech.com/upload/youtubeuseruploads/YouTubeUserUploads-source.zip
It won't be the best code you'll ever see, but it does the job for the task at hand.
YouTubeUserUploads.exe <username> [file]
If [file] is not given, a file links.txt will be created in the current directory.
That would create (or overwrite) a text file called links.txt and write the links to my three currently uploaded videos at the time of writing:
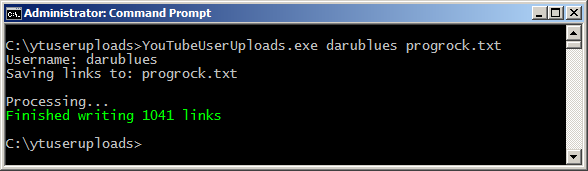
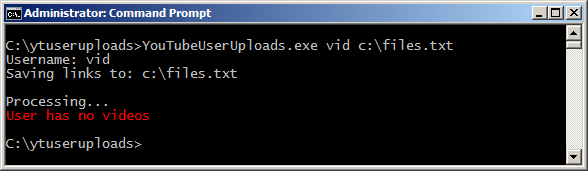
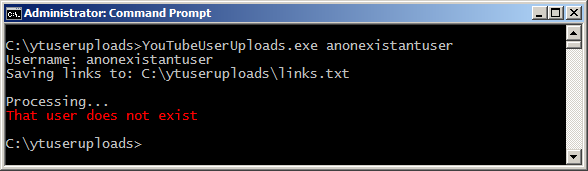
Download
Since this was such a small project, I didn't see fit to have a github repository for it so I just uploaded it to my hosting server.Download the exe (release) file from: http://dreasgrech.com/upload/youtubeuseruploads/YouTubeUserUploads.exe
And the source: http://dreasgrech.com/upload/youtubeuseruploads/YouTubeUserUploads-source.zip
It won't be the best code you'll ever see, but it does the job for the task at hand.
Usage
If [file] is not given, a file links.txt will be created in the current directory.
Demo
Here's an example run using my YouTube username:C:\YouTubeUserUploads.exe agnt666 uploads.txt Username: agnt666 Saving links to: uploads.txt Processing... Finished writing 3 links
That would create (or overwrite) a text file called links.txt and write the links to my three currently uploaded videos at the time of writing:
http://www.youtube.com/watch?v=rsXnEpWyeEg http://www.youtube.com/watch?v=sxBiD7u7nx8 http://www.youtube.com/watch?v=Ri2SHGZaSqs
Screenshots
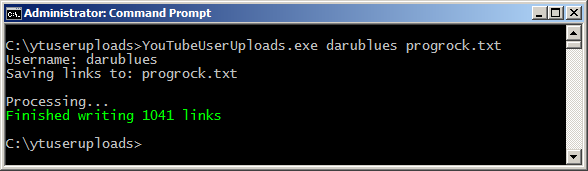
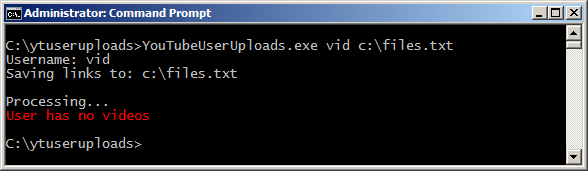
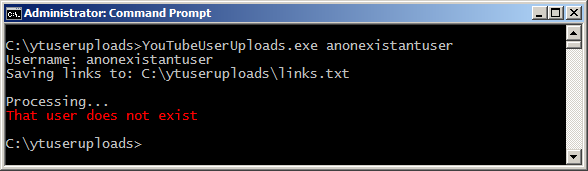
Thursday, September 8, 2011
The Monty Hall game
After my last post on simulating the Monty Hall problem, I decided to go a step further and actually write the game itself. Since I wrote the simulation using JavaScript, I kept at it by writing the game using JavaScript by making use of the canvas element.
You can play the game here and find the source on Github: https://github.com/dreasgrech/The-Monty-Hall-Game.
The code leaves much to be desired but since this was a quick weekend project (...although some tweaks did spill over to the rest of the week), I didn't worry too much about it.
You can play the game here and find the source on Github: https://github.com/dreasgrech/The-Monty-Hall-Game.
The code leaves much to be desired but since this was a quick weekend project (...although some tweaks did spill over to the rest of the week), I didn't worry too much about it.
Screenshots
Posted by
Andreas Grech
at
5:26 PM
The Monty Hall game
2011-09-08T17:26:00+02:00
Andreas Grech
Game Development|Javascript|Projects|
Comments


Labels:
Game Development,
Javascript,
Projects
Saturday, September 3, 2011
Simulating the Monty Hall problem
You're on a game show and begin the game by having three doors in front of you. Behind one of the doors, lies the prize. You don't know which door is the winning door but the game show host knows.
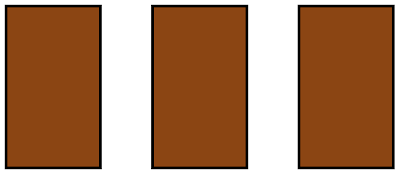
You now randomly choose the door which you think has the prize behind it, but the door doesn't open for the time being. In this case, we are going to choose the middle door.
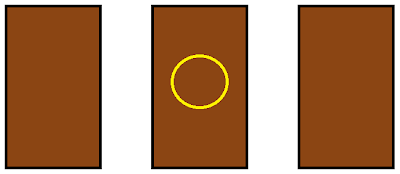
The game show host must now open one of the two remaining doors, and the door he chooses must not be the winning door. For this example, the game show host opens the door on the right.
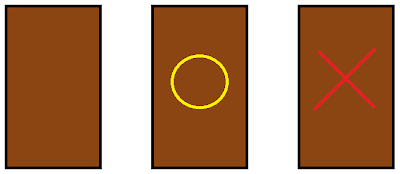
The host now asks you if you want to stick with your first choice or switch to the remaining door.
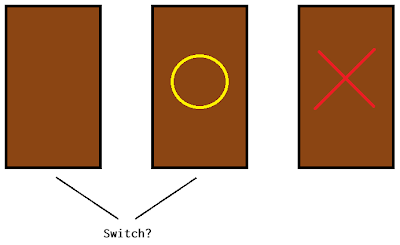
Is it to your advantage to change your choice?
To test this out, I wrote some code using JavaScript to simulate playing a number of games and supplying me with the results of how many times it won when switching or not switching the chosen door upon given the choice. The initial guesses are all random.
These results show how the number of times you win when switching the door is approximately double the number of times you win when not switching the door.
This may seem counter intuitive at first as you may think that there's a 1/3 chance of winning, but as you can see from the above results, that's actually not the case. In fact, switching the door wins twice as often staying.
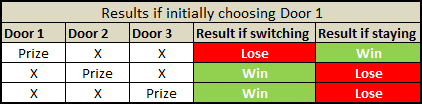
The same goes for when initially choosing Door 2 or Door 3:
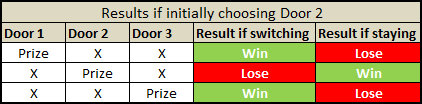
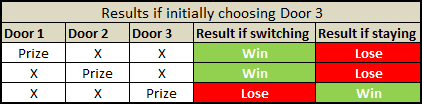
Notice how, irrespective of which door you initially choose, you always have 2/3 chance of winning if you then switch.
I've added this piece of code to the Monty Hall problem at the Rosetta Code website.
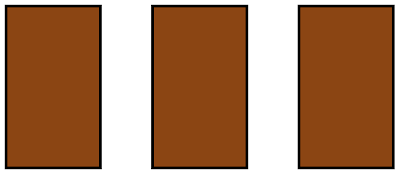
You now randomly choose the door which you think has the prize behind it, but the door doesn't open for the time being. In this case, we are going to choose the middle door.
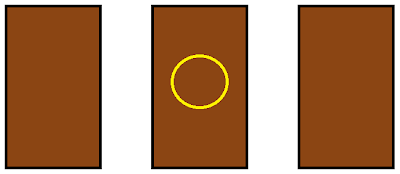
The game show host must now open one of the two remaining doors, and the door he chooses must not be the winning door. For this example, the game show host opens the door on the right.
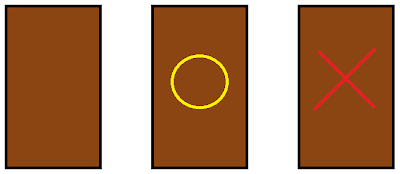
The host now asks you if you want to stick with your first choice or switch to the remaining door.
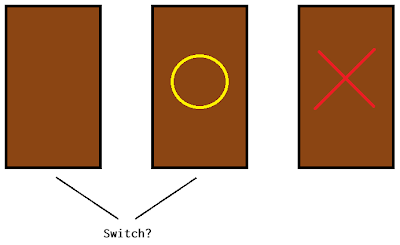
To test this out, I wrote some code using JavaScript to simulate playing a number of games and supplying me with the results of how many times it won when switching or not switching the chosen door upon given the choice. The initial guesses are all random.
Results
The following is the output for 5 runs with 10,000 games each:Playing 10000 games Wins when not switching door 3317 Wins when switching door 6738 Playing 10000 games Wins when not switching door 3376 Wins when switching door 6735 Playing 10000 games Wins when not switching door 3287 Wins when switching door 6726 Playing 10000 games Wins when not switching door 3298 Wins when switching door 6664 Playing 10000 games Wins when not switching door 3302 Wins when switching door 6674
These results show how the number of times you win when switching the door is approximately double the number of times you win when not switching the door.
This may seem counter intuitive at first as you may think that there's a 1/3 chance of winning, but as you can see from the above results, that's actually not the case. In fact, switching the door wins twice as often staying.
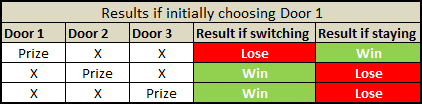
The same goes for when initially choosing Door 2 or Door 3:
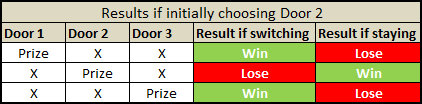
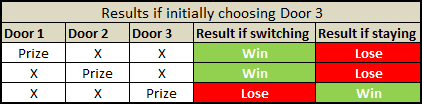
Adapted from [Vos Savant, 1990]
Notice how, irrespective of which door you initially choose, you always have 2/3 chance of winning if you then switch.
The code
var totalGames = 10000, selectDoor = function () { return Math.floor(Math.random() * 3); // Choose a number from 0, 1 and 2. }, games = (function () { var i = 0, games = []; for (; i < totalGames; ++i) { games.push(selectDoor()); // Pick a door which will hide the prize. } return games; }()), play = function (switchDoor) { var i = 0, j = games.length, winningDoor, randomGuess, totalTimesWon = 0; for (; i < j; ++i) { winningDoor = games[i]; randomGuess = selectDoor(); if ((randomGuess === winningDoor && !switchDoor) || (randomGuess !== winningDoor && switchDoor)) { /* * If I initially guessed the winning door and didn't switch, * or if I initially guessed a losing door but then switched, * I've won. * * The only time I lose is when I initially guess the winning door * and then switch. */ totalTimesWon++; } } return totalTimesWon; }; /* * Start the simulation */ console.log("Playing " + totalGames + " games"); console.log("Wins when not switching door", play(false)); console.log("Wins when switching door", play(true));
I've added this piece of code to the Monty Hall problem at the Rosetta Code website.
Posted by
Andreas Grech
at
7:57 PM
Simulating the Monty Hall problem
2011-09-03T19:57:00+02:00
Andreas Grech
Javascript|Mathematics|
Comments


Labels:
Javascript,
Mathematics
Friday, August 26, 2011
Balls to the Canvas!
To play around a bit with the canvas element, I decided to write this:
You can increase or decrease the number of balls on screen using the UP and DOWN arrows respectively.
View it in action
The source is here: https://github.com/dreasgrech/balls
The initial value of the hue is randomly chosen at the beginning.
Since collision detection is only applied once the balls hit the edges of the browser window and the edges are axes aligned, I'm simply inverting the part of the velocity's depending to which edge is hit.
If the ball hits either the left or right edge, the x is inverted and if the ball hits the top or bottom edge, the y is inverted.
You can increase or decrease the number of balls on screen using the UP and DOWN arrows respectively.
The source is here: https://github.com/dreasgrech/balls
Some implementation details
The background
To implement the colorful background, I'm using a cylindrical-coordinate representation of the RGB color model, known as HSV (Hue Saturation Value). Keeping the saturation and value to a constant 100 (max), I'm iterating through values 0-360 for the hue thus achieving the blending of colors. When the hue reaches 360, I reset it back to 0 and the cycle starts over.The initial value of the hue is randomly chosen at the beginning.
Balls
All of the balls' attributes are randomly generated i.e. the initial position, size, color, speed and angle.Since collision detection is only applied once the balls hit the edges of the browser window and the edges are axes aligned, I'm simply inverting the part of the velocity's depending to which edge is hit.
If the ball hits either the left or right edge, the x is inverted and if the ball hits the top or bottom edge, the y is inverted.
Resizing the window
I'm hooking to window.onresize so that once the browser window is resized, I adjust the width of the canvas appropriately and also remove the balls that are now out of screen.
Posted by
Andreas Grech
at
7:56 PM
Balls to the Canvas!
2011-08-26T19:56:00+02:00
Andreas Grech
Javascript|Projects|
Comments


Labels:
Javascript,
Projects
Saturday, May 14, 2011
Solving systems of linear algebra with Wolfram Alpha
I recently needed to verify an answer to some problems I was solving at the time, and for that, I used Wolfram Alpha.
Say we want to graph the following system of equations:
The syntax would be:
The general format would be:
And the search engine will provide you with something like this:
Say we want to graph the following system of equations:
The syntax would be:
solve 2x + y = 3 , x - 3y = 0
The general format would be:
solve <equation 1>, <equation 2>, ... , <equation n>
And the search engine will provide you with something like this:
Posted by
Andreas Grech
at
8:59 PM
Solving systems of linear algebra with Wolfram Alpha
2011-05-14T20:59:00+02:00
Andreas Grech
Mathematics|
Comments


Labels:
Mathematics
Saturday, April 2, 2011
Writing brainfuck in Bitmap format
Inspired by this beautiful answer from Stack Overflow, I decided I'd do something similar.
The following is a bitmap image I drew in Paint visually showing a working brainfuck program:
Download the image, feed it to your brainfuck compiler and then execute the compiled executable.
If everything goes well, you should get something like this:
The following is a bitmap image I drew in Paint visually showing a working brainfuck program:
Download the image, feed it to your brainfuck compiler and then execute the compiled executable.
If everything goes well, you should get something like this:
Sunday, March 27, 2011
Finding the Answer (ab)using JavaScript
This is my little tribute to Douglas Adams (and also my first go at obfuscation):
If the layout screwed up, here's how it should look like:
Click here to run it.
tl;dr: it was fun.
Now basically, the code does 'nothing' except alert the number 42. Actually, the majority of the code is doing a calculation to come up with the number 42, and then alert it.
Removing a single character from the above code will either invalidate the output or invalidate the code itself with a syntax error (except from that redundant whitespace on the last line)...and that was actually quite tricky to do (and may not have fully achieved since it wasn't the plan from the beginning), but was part of the fun in creating this.
I was actually writing a script that would run through my code removing a single character each time and then running (eval) the script, but I didn't finish it because as it turns out, complicating shit can be pretty fun so I switched back to this.
But the major part of the fun was finding creative ways of complicating things. Yup, complicating things! It felt refreshingly good trying to, for a change, obscure the code as much as possible given that every character is needed for the ultimate outcome.
For example, let's say I had a calculation which involved the number zero. Now, the way a sane programmer would represent a zero is by using the 0 literal representation. But that's obviously too boring for a project like this, so I would try and find new ways of representing the value of zero. An example would be something like +!!NaN, or maybe -{}>>1.
Of course, there's a reason why both those obscure representations evaluate to 0 and how my above code manages to compute and alert the number 42, and one way of finding out those reasons is by painstakingly analysing the spec.
If you don't have time to carefully go through those 252 pages, what you really need to grasp is JavaScript's type coercion rules due to weak typing...and then, abuse them mercilessly!
I'm not really going to get too much into JavaScript's type system in this post, but I will briefly explain why the above two examples I mentioned evaluate to 0.
Let's take -{}>>1 first. According to JavaScript's operator precedence rules, the unary negation sign is evaluated first, thus coercing {} into the special NaN value. Then the bitwise shift coerces the NaN value into a 0 (since NaN is falsy) and 0 >> 1 is 0.
Now for +!!NaN, starting with !NaN evaluating to the boolean value true because the logical not sign coerces the NaN value to the boolean value false, and the negation (logical-not) of false is true, so !!NaN evaluates to false because double negating false evaluates to true. Finally, since the + here is used as a unary operation and not binary, it coerces false to 0; and that's it!
Up until the end, I was working on a single line with no whitespace but when I was sort of ready with obfuscating, I needed to split the lines up into some shape (remotely representing something)...a task which I figured to be trivial at best. But as I came to realize, splitting the code into multiple lines without introducing errors is far from trivial.
Reason being of course that you can't just split the line in whichever point you want because naturally keywords cannot be split and other restrictions of the language syntax restrict you from splitting certain constructs directly into multiple lines.
For example, say you have function(){return}, you can't for example split in the middle of the word function:
fun
ction(){return}
That will not compile, and neither will compile if you split the return keyword; you can split at any other part of the line though.
But I actually had the most trouble splitting property calls. Say you had to split [].constructor.prototype; now that's a bitch because of the two relatively long keywords in the expression.
How did I get around this? Simple. I switched from using dot-notation to subscript-notation, and since strings can be successfully split into multiple lines, it was much easier:
[]['constructor']['prototype']
Now if we wanted to split:
[]['construc\
tor']['prototype']
To split strings into multiple lines in JavaScript, just add the backslash symbol to the end of the unterminated string literal, and now, your splitting task is much easier.
Although using the subscript-notation made the lines easier to split, it introduced a new problem.
Say I have ['ab'] and I need to split between the ' and the a characters.
You can't split like this:
['
ab']
because now you have an unterminated string, which results in an error; but you also can't do this:
['\
ab']
because although it runs successfully, you have now added an extra character (\) to the previous line and that would invalidate your box-line shape of the code. And of course, can't also do this:
[
'ab']
because, as mentioned previously, although it runs, you have now removed a character from the previous line which would invalidate the box.
This one was a bit more annoying to deal with because, to my knowledge, there is no way around it...but still, this was all part of the challenge involved and it was fun.
vI=0x2A;(o=eval)((r=unescape)("%5F%5f%"+vI/+(+vI+'')[1]%6+"D%36%3b"))+o(r("%"+__+"1\ %6"+(ww=String["fromCharCode"])(69)+"%73%77%65%72%3D")+((~~[]+011>>!~~~(J=Math)[""]+ (Jan=isNaN)(+/\//[+[]*+-"1"]+""[-1])*~!-+(___=__/2)+2^!+!J['round'](![H=undefined]+1 +o((J+1).substr(!+(B=alert)+-!B+7<<.9-1,!/^/.$-~255>>(2*!o('('+(F='function(')+'ema\ cs){if((dreas=vI)>emacs){always=1}}(vI-5))')-~1*1-(+!(function(){o(arguments[__['co\ nstructor'].prototype*9]+"='"+arguments[0]+"'")}('$'+__))))*2)+"Magnum/* */"[!+''+7] +".PI")^!H*!Jan(Jan)-+2+1))<<(!0x0-[010][dreas%2]/2+(4&4)+o("'.'+o(((1|__^4*2)+\"\"\ )[1])")<<+!+(c=NaN)<<1^~+!c*-___+(+J['floor'](~2)^-4))|/**/!![,]["le"+($$$=(parseInt +2))[2]+"g"+$$$[4]+"h"]/**/*(o("o('~1+010+/*~*/~([][0,+$6[1]+2]-always|!{a:1}[0]+24\ <<1)%15<<~!J.ceil.call.apply(J.ceil,[1..toString()])+1')")+0xE^3<<1)>>+!o('('+F+'){\ _:if(!(_=!1)){return}}())')>>!null+_));o(r(["%42",(o+'')[13],answer,(r+'')[18],ww(59 )]. join ('')))
If the layout screwed up, here's how it should look like:
Click here to run it.
Why?
tl;dr: it was fun.
Now basically, the code does 'nothing' except alert the number 42. Actually, the majority of the code is doing a calculation to come up with the number 42, and then alert it.
Removing a single character from the above code will either invalidate the output or invalidate the code itself with a syntax error (except from that redundant whitespace on the last line)...and that was actually quite tricky to do (and may not have fully achieved since it wasn't the plan from the beginning), but was part of the fun in creating this.
I was actually writing a script that would run through my code removing a single character each time and then running (eval) the script, but I didn't finish it because as it turns out, complicating shit can be pretty fun so I switched back to this.
But the major part of the fun was finding creative ways of complicating things. Yup, complicating things! It felt refreshingly good trying to, for a change, obscure the code as much as possible given that every character is needed for the ultimate outcome.
How?
For example, let's say I had a calculation which involved the number zero. Now, the way a sane programmer would represent a zero is by using the 0 literal representation. But that's obviously too boring for a project like this, so I would try and find new ways of representing the value of zero. An example would be something like +!!NaN, or maybe -{}>>1.
Of course, there's a reason why both those obscure representations evaluate to 0 and how my above code manages to compute and alert the number 42, and one way of finding out those reasons is by painstakingly analysing the spec.
If you don't have time to carefully go through those 252 pages, what you really need to grasp is JavaScript's type coercion rules due to weak typing...and then, abuse them mercilessly!
I'm not really going to get too much into JavaScript's type system in this post, but I will briefly explain why the above two examples I mentioned evaluate to 0.
Let's take -{}>>1 first. According to JavaScript's operator precedence rules, the unary negation sign is evaluated first, thus coercing {} into the special NaN value. Then the bitwise shift coerces the NaN value into a 0 (since NaN is falsy) and 0 >> 1 is 0.
Now for +!!NaN, starting with !NaN evaluating to the boolean value true because the logical not sign coerces the NaN value to the boolean value false, and the negation (logical-not) of false is true, so !!NaN evaluates to false because double negating false evaluates to true. Finally, since the + here is used as a unary operation and not binary, it coerces false to 0; and that's it!
Splitting it up
Up until the end, I was working on a single line with no whitespace but when I was sort of ready with obfuscating, I needed to split the lines up into some shape (remotely representing something)...a task which I figured to be trivial at best. But as I came to realize, splitting the code into multiple lines without introducing errors is far from trivial.
Reason being of course that you can't just split the line in whichever point you want because naturally keywords cannot be split and other restrictions of the language syntax restrict you from splitting certain constructs directly into multiple lines.
For example, say you have function(){return}, you can't for example split in the middle of the word function:
fun
ction(){return}
That will not compile, and neither will compile if you split the return keyword; you can split at any other part of the line though.
But I actually had the most trouble splitting property calls. Say you had to split [].constructor.prototype; now that's a bitch because of the two relatively long keywords in the expression.
How did I get around this? Simple. I switched from using dot-notation to subscript-notation, and since strings can be successfully split into multiple lines, it was much easier:
[]['constructor']['prototype']
Now if we wanted to split:
[]['construc\
tor']['prototype']
To split strings into multiple lines in JavaScript, just add the backslash symbol to the end of the unterminated string literal, and now, your splitting task is much easier.
Although using the subscript-notation made the lines easier to split, it introduced a new problem.
Say I have ['ab'] and I need to split between the ' and the a characters.
You can't split like this:
['
ab']
because now you have an unterminated string, which results in an error; but you also can't do this:
['\
ab']
because although it runs successfully, you have now added an extra character (\) to the previous line and that would invalidate your box-line shape of the code. And of course, can't also do this:
[
'ab']
because, as mentioned previously, although it runs, you have now removed a character from the previous line which would invalidate the box.
This one was a bit more annoying to deal with because, to my knowledge, there is no way around it...but still, this was all part of the challenge involved and it was fun.
Posted by
Andreas Grech
at
3:26 PM
Finding the Answer (ab)using JavaScript
2011-03-27T15:26:00+02:00
Andreas Grech
Javascript|
Comments


Labels:
Javascript
Saturday, February 26, 2011
Adapting to Farseer Physics Engine's Meter-Kilogram-Second (MKS) system of units
Recently switched to version 3.x of Farseer Physics Engine and have been experiencing slow simulations since? Can't get anything to accelerate past a certain point because everything looks like it's floating in a damn aquarium? Are you frustrated and tearing your hair out because you think this new version "sucks" !? Don't worry, you're not alone.
Now, take a deep breath and carry on reading...(tl;dr: demo)
Starting with 3.x, FPE now uses the MKS (Meter-Kilogram-Second) system of units and this has been the source of confusion for both people who were working with version < 3 and also people who were new to FPE.
Note: Other people have already written about this topic but I feel that, since this has been a major source of confusion for many, it hasn't been given the attention it requires.
To begin with, let's be naive and try to create a Body like such:
In the Draw method, I (naively) draw the texture at the body's position as follows:
What I'm doing above (or what at least I think I'm doing) is create a 200x100 pixel rectangular body positioned at (200, 300) pixels on the screen and then drawing it at wherever the body is at the current moment, starting from our initial (300, 400).
But because now Farseer Physics Engine uses the MKS system of units, what we are actually doing is creating a 200m wide and a 100m high rectangle rectangle positioned 200 meters from the right and 100 meters from the top! And Box2D (since Farseer is based on Box2D) tells us that we should keep our bodies (especially if they move) in the 0.1 - 10 meter range.
This means that we now have to split the way we position physics objects on screen and the way we draw their corresponding textures.
To accomplish this, we can use the ConvertUnits class found under Samples\Samples XNA\Samples XNA\ScreenSystem.
ConvertUnits is a helper class that lets us switch between display units (pixels) and simulation units (MKS) easily.
So let's go back to that previous example and fix our code by first converting our display units to simulation units with ConvertUnits.ToSimUnits:
Now to draw our body on screen, we need to convert the simulation units back to display units by the appropriately named method ConvertUnits.ToDisplayUnits:
Still can't get it? No worries, because I created the simplest Farseer Physics 3 example you can find which demonstrates what I said above: https://github.com/dreasgrech/SimplestFarseerPhysics3Example
The example creates a rectangle and lets gravity do its part; that's it! I've compiled it for both the Windows and the Windows Phone 7 XNA versions.
Another note: Since FPE is currently undergoing the upgrade to 3.3, the above code example can break if used with a different version of the engine. I've compiled the example with the version from Changeset #85352.
Now, take a deep breath and carry on reading...(tl;dr: demo)
Starting with 3.x, FPE now uses the MKS (Meter-Kilogram-Second) system of units and this has been the source of confusion for both people who were working with version < 3 and also people who were new to FPE.
Note: Other people have already written about this topic but I feel that, since this has been a major source of confusion for many, it hasn't been given the attention it requires.
To begin with, let's be naive and try to create a Body like such:
float width = 200f, height = 100f, density = 10f; Vector2 position = new Vector2(300, 400); Body body = BodyFactory.CreateRectangle(world, width, height, density, position);
In the Draw method, I (naively) draw the texture at the body's position as follows:
spriteBatch.Draw(texture, body.Position, Color.White);
What I'm doing above (or what at least I think I'm doing) is create a 200x100 pixel rectangular body positioned at (200, 300) pixels on the screen and then drawing it at wherever the body is at the current moment, starting from our initial (300, 400).
But because now Farseer Physics Engine uses the MKS system of units, what we are actually doing is creating a 200m wide and a 100m high rectangle rectangle positioned 200 meters from the right and 100 meters from the top! And Box2D (since Farseer is based on Box2D) tells us that we should keep our bodies (especially if they move) in the 0.1 - 10 meter range.
This means that we now have to split the way we position physics objects on screen and the way we draw their corresponding textures.
To accomplish this, we can use the ConvertUnits class found under Samples\Samples XNA\Samples XNA\ScreenSystem.
ConvertUnits is a helper class that lets us switch between display units (pixels) and simulation units (MKS) easily.
So let's go back to that previous example and fix our code by first converting our display units to simulation units with ConvertUnits.ToSimUnits:
float width = ConvertUnits.ToSimUnits(200f), height = ConvertUnits.ToSimUnits(100f), density = 10f; Vector2 position = ConvertUnits.ToSimUnits(300, 400); // ToSimUnits has an overload which returns a vector given two floats. Body body = BodyFactory.CreateRectangle(world, width, height, density, position);
Now to draw our body on screen, we need to convert the simulation units back to display units by the appropriately named method ConvertUnits.ToDisplayUnits:
spriteBatch.Draw(texture, ConvertUnits.ToDisplayUnits(body.Position), Color.White);
Still can't get it? No worries, because I created the simplest Farseer Physics 3 example you can find which demonstrates what I said above: https://github.com/dreasgrech/SimplestFarseerPhysics3Example
The example creates a rectangle and lets gravity do its part; that's it! I've compiled it for both the Windows and the Windows Phone 7 XNA versions.
Another note: Since FPE is currently undergoing the upgrade to 3.3, the above code example can break if used with a different version of the engine. I've compiled the example with the version from Changeset #85352.
Posted by
Andreas Grech
at
12:42 AM
Adapting to Farseer Physics Engine's Meter-Kilogram-Second (MKS) system of units
2011-02-26T00:42:00+01:00
Andreas Grech
Farseer Physics Engine|Game Development|Windows Phone 7|XNA|
Comments


Labels:
Farseer Physics Engine,
Game Development,
Windows Phone 7,
XNA
Sunday, February 13, 2011
StringEvolver: Evolving strings with a genetic algorithm
This is my first attempt at experimenting with a genetic algorithm. The following is an application that evolves a string given a destination to converge to.
Here is an example run:
A value between 0-1
The mutation rate determines the probability of how often a selected chromosome mutates. Mutation is very important in a genetic algorithm because it helps in keeping diversity in the population, thus avoid local minima which can slow or even halt further evolution.
The application currently uses a mutation operator called Single Point Mutation. For our current application, it works by randomly selecting a point in the string and switching the character at that point to another random character.
As an example, say the chromosome to be mutated currently contains the string "aBcdE". The single point mutation operator then randomly chooses position 2 (0-based) and replaces the character 'c' with the randomly chosen character 'W'.
This way, the chromosome "aBcdE" has now been mutated to "aBWdE".
-s, --crossover=VALUE
A value between 0-1
The crossover rate determines how often two selected chromosomes are sexually combined to produce two separate offspring.
For this application, there are two available crossover operations: One Point Crossover and Two Point Crossover. These operators are discussed further in the ctype argument.
-e, --elitism=VALUE
A value between 0-1
The elitism rate determines the number of the fittest solutions that are directly (untouched) transferred to the advancing population.
Elitism can help in preventing the potential loss of possibly good solutions and can lead to quicker convergence.
-c, --crcount=VALUE
A value greater than 1
The chromosome count determines the number of chromosomes that each population will have. The greater the number of chromosomes, the more time a population takes to advance to the next.
--fitness=VALUE
VALUE = sum, levenshtein or hamming
The fitness calculator determines which algorithm is used to calculate how fit a given chromosome is.
There are currently three fitness calculators to choose from:
Sum
The sum calculator calculates the ASCII difference between each character of the target strings.
The Levenshtein distance between two strings is the minimum number of edits needed to transform one string into the other. The transformation can include insertion, deletion and substitution. This distance is also referred to as the edit distance.
The Hamming distance between two strings of equal length is the number of positions at which the corresponding symbols are different. The reason Hamming distance requires the strings to be of equal length is because unlike the Levenshtein distance, the Hamming distance only allows substitutions.
As an example, the Hamming distance between "janica" and "jenixo" is 3.
--ctype=VALUE
VALUE = one or two
The crossover type determines which crossover operator is to be used when sexually combining two chromosomes.
There are currently two available methods to choose from:
One
The One-Point Crossover method chooses a random point (called a locus) in the string and all the data beyond that point in either chromosome is swapped between the parent chromosomes. The resulting two chromosomes are the children.
Two
The Two-Point Crossover method is very similar to the One-Point Crossover method but this one chooses two random points along the string rather than one. The data between the two points is swapped and the resulting chromosomes are the children.
-t, --truncate=VALUE
0 < VALUE <= 1
The Truncation value determines how many chromosomes of the current population should be kept and used for the selection, crossover and mutation operations. Note that before the truncation is performed, the chromosomes in the population are sorted in descending order by their fitness, so the fittest chromosomes are kept after the truncation.
A value of 1 uses all of the chromosomes and a value of 0.5 uses half of the population (the better half, of course)
Here is an example run:
> StringEvolver -m 0.25 -s 0.6 -c 2000 -e 0.1 --fitness=hamming --ctype=one -t 0.3 "Charles Darwin was right!" Evolution Destination: Charles Darwin was right! Mutation Rate: 25% Crossover Rate: 60% Truncation Rate: 30% Chromosomes / population: 2000 Elitism / population: 200 (0.1%) Fitness Calculator: Hamming Distance Crossover Type: One Point 1: L<eI`Y@ D#raF'JKJ7DUAg"GR 2: L<eI`Y@ D#raF'JKJ7DUAg"GR 3: chNOLe"5]={Iiyrgk*,rEpE/! 4: L<eI`Y@ D#ra nR$C1SrYqha+ 5: L<eI`Y@ D#ra nR$C1SrYqha+ 6: lpa5`Y@ D#ra nR$C8y.iqrt5 7: C]Y&hJ1 %aRwi< aaB,r_Zh68 8: 1s[r.ts %aGwi< _a"=rjXyN! 9: chNOlFsyD#rwi< }aB,r_Zh68 10: chNO9e" D#rwi< }aB=rPgh6! 11: chNO9e" D#rwi< }aB=rPgh6! 12: 1s[r.Ks D#ra n}way rAght! 13: C}hplFs D#rwi.}way rPgh6! 14: C'aclos Da{]inrwas r.ghx! 15: C'aclos Da{]inrwas r.ghx! 16: ChNrlFs Da>win}was `gha! 17: ChNrlFs Da>winlwas r;gh6! 18: Ch[rlFs Da>wi< was rAght! 19: Chacle@ D#rwin was rjght! 20: Chacle@ D#rwin was rjght! 21: ChNrles Darwin}was rAght! 22: ChNrles Darwin}was rAght! 23: Charles Darwin}was r`ght! 24: Charles Darwin}was r`ght! 25: Charles Darwin was rAght! 26: Charles Darwin was rAght! 27: Charles Darwin was rAght! 28: Charles Darwin was rAght! 29: Charles Darwin was rAght! 30: Charles Darwin was right! Found in 30 generations Time Taken: 00:00:00.4730271
Source
You can download the source from github: https://github.com/dreasgrech/StringEvolverCommand Line arguments
-m, --mutation=VALUEA value between 0-1
The mutation rate determines the probability of how often a selected chromosome mutates. Mutation is very important in a genetic algorithm because it helps in keeping diversity in the population, thus avoid local minima which can slow or even halt further evolution.
The application currently uses a mutation operator called Single Point Mutation. For our current application, it works by randomly selecting a point in the string and switching the character at that point to another random character.
As an example, say the chromosome to be mutated currently contains the string "aBcdE". The single point mutation operator then randomly chooses position 2 (0-based) and replaces the character 'c' with the randomly chosen character 'W'.
This way, the chromosome "aBcdE" has now been mutated to "aBWdE".
-s, --crossover=VALUE
A value between 0-1
The crossover rate determines how often two selected chromosomes are sexually combined to produce two separate offspring.
For this application, there are two available crossover operations: One Point Crossover and Two Point Crossover. These operators are discussed further in the ctype argument.
-e, --elitism=VALUE
A value between 0-1
The elitism rate determines the number of the fittest solutions that are directly (untouched) transferred to the advancing population.
Elitism can help in preventing the potential loss of possibly good solutions and can lead to quicker convergence.
-c, --crcount=VALUE
A value greater than 1
The chromosome count determines the number of chromosomes that each population will have. The greater the number of chromosomes, the more time a population takes to advance to the next.
--fitness=VALUE
VALUE = sum, levenshtein or hamming
The fitness calculator determines which algorithm is used to calculate how fit a given chromosome is.
There are currently three fitness calculators to choose from:
Sum
The sum calculator calculates the ASCII difference between each character of the target strings.
public override double CalculateFitness(Chromosome ch) { var distanceToTarget = Target.Select((t, i) => Math.Abs(t - ch.Value[i])).Sum(); return 1.0 / distanceToTarget; }Levenshtein Distance
The Levenshtein distance between two strings is the minimum number of edits needed to transform one string into the other. The transformation can include insertion, deletion and substitution. This distance is also referred to as the edit distance.
public override double CalculateFitness(Chromosome ch) { return 1.0 / LevenshteinDistance(ch.Value, Target); }Hamming Distance
The Hamming distance between two strings of equal length is the number of positions at which the corresponding symbols are different. The reason Hamming distance requires the strings to be of equal length is because unlike the Levenshtein distance, the Hamming distance only allows substitutions.
As an example, the Hamming distance between "janica" and "jenixo" is 3.
public override double CalculateFitness(Chromosome ch) { if (ch.Value.Length != Target.Length) { return 1.0 / double.PositiveInfinity; } var difference = 0; for (int i = 0; i < Target.Length; i++) { if (ch.Value[i] != Target[i]) { difference++; } } return 1.0 / difference; }
--ctype=VALUE
VALUE = one or two
The crossover type determines which crossover operator is to be used when sexually combining two chromosomes.
There are currently two available methods to choose from:
One
The One-Point Crossover method chooses a random point (called a locus) in the string and all the data beyond that point in either chromosome is swapped between the parent chromosomes. The resulting two chromosomes are the children.
public Tuple<Chromosome, Chromosome> Crossover(Chromosome c1, Chromosome c2) { var locus = random.Next(0, c1.Value.Length + 1); string ch1 = c1.Value.Substring(0, locus) + c2.Value.Substring(locus), ch2 = c2.Value.Substring(0, locus) + c1.Value.Substring(locus); return new Tuple<Chromosome, Chromosome>(new Chromosome(ch1, fitnessCalculator), new Chromosome(ch2, fitnessCalculator)); }
Two
The Two-Point Crossover method is very similar to the One-Point Crossover method but this one chooses two random points along the string rather than one. The data between the two points is swapped and the resulting chromosomes are the children.
public Tuple<Chromosome, Chromosome> Crossover(Chromosome c1, Chromosome c2) { int locus1 = random.Next(0, c1.Value.Length), locus2 = random.Next(locus1, c1.Value.Length), distance = locus2 - locus1; string ch1 = c1.Value.Substring(0, locus1) + c2.Value.Substring(locus1, distance) + c1.Value.Substring(locus2), ch2 = c2.Value.Substring(0, locus1) + c1.Value.Substring(locus1, distance) + c2.Value.Substring(locus2); return new Tuple<Chromosome, Chromosome>(new Chromosome(ch1, fitnessCalculator), new Chromosome(ch2, fitnessCalculator)); }
-t, --truncate=VALUE
0 < VALUE <= 1
The Truncation value determines how many chromosomes of the current population should be kept and used for the selection, crossover and mutation operations. Note that before the truncation is performed, the chromosomes in the population are sorted in descending order by their fitness, so the fittest chromosomes are kept after the truncation.
A value of 1 uses all of the chromosomes and a value of 0.5 uses half of the population (the better half, of course)
Posted by
Andreas Grech
at
8:14 PM
StringEvolver: Evolving strings with a genetic algorithm
2011-02-13T20:14:00+01:00
Andreas Grech
C#|Genetic Algorithms|Projects|
Comments


Labels:
C#,
Genetic Algorithms,
Projects
Subscribe to:
Posts (Atom)