To make use of the keypad, I used Arduino's keypad library.
I connected the keypad to the Arduino as follows:
Keypad Pin | Arduino Pin |
---|---|
1 | 7 |
2 | 5 |
3 | 8 |
4 | 2 |
5 | 6 |
6 | 3 |
7 | 4 |
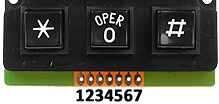
To use in your code, make sure you include it:
#include <Keypad.h>
You also need to map out the keys like such:
const byte ROWS = 4; //four rows const byte COLS = 3; //three columns char keys[ROWS][COLS] = { { '1','2','3' } , { '4','5','6' } , { '7','8','9' } , { '*','0','#' } }; //The below numbers correspond to the Arduino Pins byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
Afterwards, instantiate the Keypad:
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
Then to get the key pressed, it's just as simple as this:
char key = keypad.getKey();